How To Upload Files In Chunks In Javascript
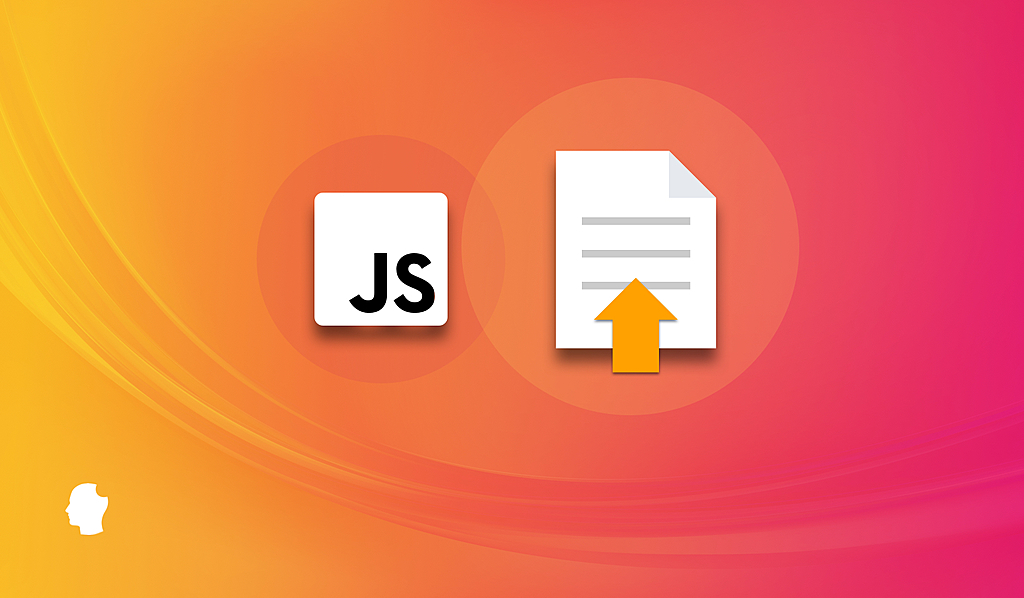
If you've spent any amount of fourth dimension messing with PHP config files to get a file to upload, you know that uploading large files tin be a real hurting. You take to find the loaded php.ini
file, edit the upload_max_filesize
and post_max_size
settings, and hope that you never have to modify servers and do all of this over again.
I ran into this problem while working on WP Migrate DB Pro. One of its features is the ability to upload and import an SQL file. WP Migrate DB Pro is used on a ton of servers, so I needed to create an upload tool that can handle large files without hitting upload limits.
Meet the JavaScript FileReader API. It'due south an easy way to read and process a file straight in the browser. The JavaScript FileReader API now has major browser support including Chrome, Firefox, Safari, and even Cyberspace Explorer 10.
With that in mind, allow'southward create a bones (no Vue or React here!) JavaScript file upload example in a WordPress plugin to acquire about the FileReader API.
Getting Started
Since the FileReader API is baked into JavaScript, the HTML side of things is easy and relies on a basic HTML form with a file input element:
<grade> <input type="file" name="dbi_import_file" /><br><br> <input blazon="submit" value="Upload" /> </grade>
To make things easier we're going to create a small grade to contain most of our code. Nosotros'll place the higher up form inside a WordPress dashboard widget:
<?php /** * Plugin Name: DBI File Uploader * Description: Upload big files using the JavaScript FileReader API * Author: Delicious Brains Inc * Version: 1.0 */ class DBI_File_Uploader { public role __construct() { add_action( 'admin_enqueue_scripts', array( $this, 'enqueue_scripts' ) ); add_action( 'wp_dashboard_setup', array( $this, 'add_dashboard_widget' ) ); add_action( 'wp_ajax_dbi_upload_file', assortment( $this, 'ajax_upload_file' ) ); } public function enqueue_scripts() { $src = plugins_url( 'dbi-file-uploader.js', __FILE__ ); wp_enqueue_script( 'dbi-file-uploader', $src, array( 'jquery' ), false, true ); wp_localize_script( 'dbi-file-uploader', 'dbi_vars', array( 'upload_file_nonce' => wp_create_nonce( 'dbi-file-upload' ), ) ); } public function add_dashboard_widget() { wp_add_dashboard_widget( 'dbi_file_upload', 'DBI File Upload', assortment( $this, 'render_dashboard_widget' ) ); } public function render_dashboard_widget() { ?> <form> <p id="dbi-upload-progress">Please select a file and click "Upload" to continue.</p> <input id="dbi-file-upload" type="file" name="dbi_import_file" /><br><br> <input id="dbi-file-upload-submit" class="button push-primary" type="submit" value="Upload" /> </form> <?php } }
With the upload grade in place we should see a basic file upload class when we visit the WordPress dashboard:
Uploading the File
The HTML class doesn't do anything notwithstanding, so let'south create the dbi-file-uploader.js
file and add an event handler for the upload button. After selecting the file object and creating the FileReader object, it will call upload_file()
to starting time the read operation:
(function( $ ) { var reader = {}; var file = {}; var slice_size = 1000 * 1024; part start_upload( consequence ) { upshot.preventDefault(); reader = new FileReader(); file = document.querySelector( '#dbi-file-upload' ).files[0]; upload_file( 0 ); } $( '#dbi-file-upload-submit' ).on( 'click', start_upload ); office upload_file( offset ) { } })( jQuery );
Now we tin commencement working on the upload_file()
office that will practice most of the heavy lifting. First we grab a chunk of the selected file using the JavaScript slice()
method:
office upload_file( first ) { var next_slice = get-go + slice_size + i; var hulk = file.slice( get-go, next_slice ); }
We'll also need to add a function inside the upload_file()
function that will run when the FileReader API has read from the file.
reader.onloadend = function( outcome ) { if ( event.target.readyState !== FileReader.Done ) { return; } // At this point the file information is loaded to event.target.result };
At present we need to tell the FileReader API to read a clamper of the file content. We can do that by passing the blob of information that we created to the FileReader object:
reader.readAsDataURL( blob );
It'due south worth noting that we're using the readAsDataURL()
method of the FileReader object, not the readAsText()
or readAsBinaryString()
methods that are in the docs.
The readAsDataURL()
method is better hither since information technology is read as Base64 instead of patently text or binary data. This is important because the latter will likely run into encoding issues when sent to the server, especially when uploading anything other than basic text files. Base64 volition ordinarily but contain the A-Z
, a-z
, and 0-9
characters. It'southward besides easy to decode with PHP 🙂.
Now let's add together the AJAX call that sends the chunk to the server. That AJAX telephone call will telephone call upload_file()
again when the request has completed. Here's what upload_file()
looks like in its entirety:
function upload_file( start ) { var next_slice = start + slice_size + 1; var blob = file.slice( get-go, next_slice ); reader.onloadend = part( event ) { if ( event.target.readyState !== FileReader.DONE ) { return; } $.ajax( { url: ajaxurl, type: 'POST', dataType: 'json', cache: imitation, information: { action: 'dbi_upload_file', file_data: upshot.target.upshot, file: file.name, file_type: file.type, nonce: dbi_vars.upload_file_nonce }, error: function( jqXHR, textStatus, errorThrown ) { console.log( jqXHR, textStatus, errorThrown ); }, success: function( data ) { var size_done = start + slice_size; var percent_done = Math.floor( ( size_done / file.size ) * 100 ); if ( next_slice < file.size ) { // Update upload progress $( '#dbi-upload-progress' ).html( `Uploading File - ${percent_done}%` ); // More to upload, call office recursively upload_file( next_slice ); } else { // Update upload progress $( '#dbi-upload-progress' ).html( 'Upload Complete!' ); } } } ); }; reader.readAsDataURL( blob ); }
And that'southward information technology for the front-stop of our javascript file upload case. It'south nonetheless pretty elementary, just that should be enough to become the file upload going on the client side.
Saving Chunks Server-Side
Now that JavaScript has split up the file upward and sent information technology to the server, we need to re-assemble and salve those chunks to the filesystem. To exercise that, we're going to add the ajax_upload_file()
method to our main plugin class:
public function ajax_upload_file() { check_ajax_referer( 'dbi-file-upload', 'nonce' ); $wp_upload_dir = wp_upload_dir(); $file_path = trailingslashit( $wp_upload_dir['path'] ) . $_POST['file']; $file_data = $this->decode_chunk( $_POST['file_data'] ); if ( false === $file_data ) { wp_send_json_error(); } file_put_contents( $file_path, $file_data, FILE_APPEND ); wp_send_json_success(); } public role decode_chunk( $data ) { $data = explode( ';base64,', $information ); if ( ! is_array( $data ) || ! isset( $data[1] ) ) { return false; } $information = base64_decode( $data[ane] ); if ( ! $data ) { return false; } return $information; }
This is about as uncomplicated as it gets – the ajax_upload_file()
method does a quick nonce check and then decodes the data in decode_chunk()
. If the information can be decoded from Base64, it is added to the file and the upload continues.
With that in identify nosotros should be able to run our uploader and the file should get saved to the called path:
And that'southward it, we take a working AJAX file uploader! If you've been post-obit along and want to encounter the full lawmaking, I've uploaded it to GitHub so you lot can take a await.
Decision
I like how easy it is to create an AJAX file uploader that tin can handle large files without needing to conform any settings server-side. Information technology's always interesting when ideas that were merely pipe dreams in the past become common and greatly better today's workflows.
It's worth noting that there are several existing JavaScript libraries like FineUploader and jQuery File Upload that can also upload big files. In many cases, it makes more than sense to use existing code instead of reinventing the wheel. But it never hurts to understand what is going on backside the scenes in case you ever need to fix a issues or add a new feature.
If you're going to utilize something like this in a existent app, you should definitely look up any security problems. This could include file type validation, preventing uploads of executable files, and making sure that uploaded files have a random string in the filename. You may besides desire to add a way for users to pause or abolish the upload and come back to it later, and log any errors that come upwardly during the upload. To speed things upward, you could look at using the Residuum API instead of admin-ajax.php.
Take you ever had to handle large file uploads? If so, did y'all take a similar approach or did you practise something completely different? Allow me know in the comments below.
Source: https://deliciousbrains.com/using-javascript-file-api-to-avoid-file-upload-limits/
Posted by: grahamwhispored.blogspot.com
0 Response to "How To Upload Files In Chunks In Javascript"
Post a Comment